When it comes to Python numbers, having a strong grasp of integer and float manipulation is crucial. In this tutorial, we’ll delve into various techniques and tricks to master these fundamental aspects of Python programming.
1. Grasping Numeric Data Types
Before diving into manipulation, let’s first review the core numerical data types: integers and floats. Understanding these types is essential for effective manipulation.
x = 5 # Integer
y = 3.14 # Float
2. Foundational Arithmetic Operations
Performing arithmetic operations is the cornerstone of number manipulation. Python’s syntax makes it intuitive to work with numbers.
sum_result = x + y
diff_result = x - y
prod_result = x * y
div_result = x / y
3. Transitioning Between Numeric Forms
Converting between different numeric forms is a common task when dealing with numeric data in Python.
x_float = float(x)
y_int = int(y)
4. Precision Management
Floats can sometimes be imprecise due to the way computers represent them.
result = 0.1 + 0.2 # Might not be exactly 0.3
5. Presenting Numeric Output
Presenting numbers neatly is important for readability, especially in output.
formatted = "Value: {:.2f}".format(x)
6. Advanced Operations with NumPy
For more sophisticated numeric operations, the NumPy library is incredibly useful. Let’s explore a simple example.
import numpy as np
array = np.array([x, y, x_float])
sum_array = np.sum(array)
By mastering integer and float manipulation in Python, you’ll gain a solid foundation for working with various types of data and solving complex problems. Strengthen your Python numbers skills and elevate your programming prowess.
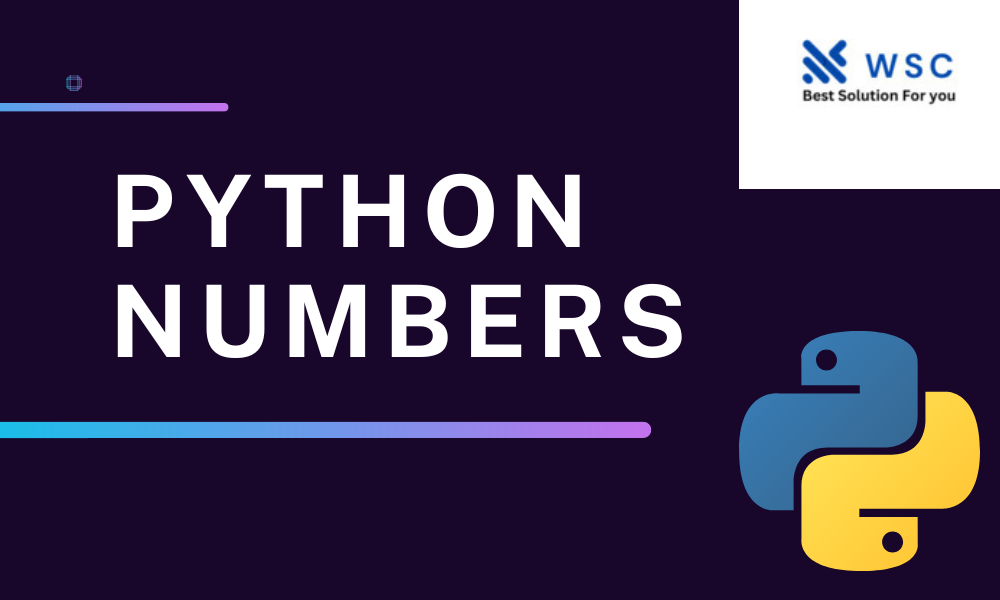
Check our tools website Word count
Check our tools website check More tutorial